Thanks to its adaptability and strong programming features, the VEX Robotics platform has become rather popular among teachers, hobbyists, and competitive teams. Understanding the DeviceURL is absolutely crucial for using the VEX Brain, especially when developing with Node.js. This post will explore DeviceURL’s definition, what is deviceurl for vex brain nodejs, interactions with the VEX Brain, and Node.js application use tips.
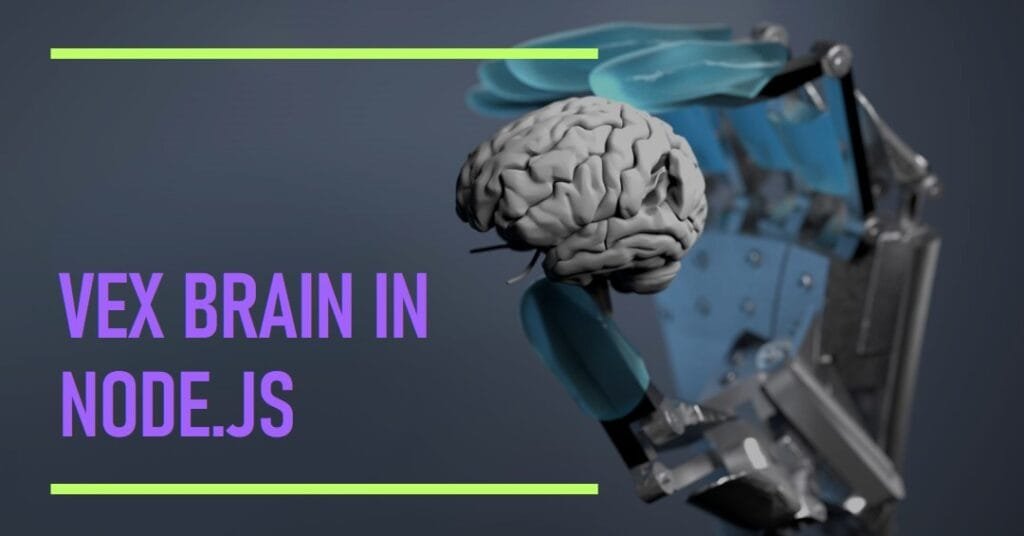
What is the VEX Brain?
The center processing unit of the VEX robotics system is the VEX Brain. Acting as the robot’s brain, it what is deviceurl for vex brain nodejs, lets different sensors, motors, and other parts communicate with one another The VEX Brain lets users build flexible robotic systems by connecting to many devices via a range of interfaces.
VEX offers many programming possibilities; among advanced users, Node.js is among the most often used one. Built on Chrome’s V8 engine, Node.js, a JavaScript runtime, is perfect for asynchronous programming and fits robotics applications needing real-time processing and speed.
What is DeviceURL?
A special identification used in the VEX Robotics system, DeviceURL links particular devices connected to the VEX Brain. Every device—including motors, sensors, or other peripherals—has a unique DeviceURL that lets developers programmatically interface with these parts.
Structure of DeviceURL
Usually following a defined format including information on the device type, port number, and other pertinent criteria, a DeviceURL in the VEX Robotics ecosystem is The usually follows:
arduino
Copy code
vex://[device_type]/[port_number]
- device_type: This refers to the type of device connected to the VEX Brain, such as a motor, sensor, or controller.
- port_number: This indicates the specific port to which the device is connected.
Examples of DeviceURL
Motor DeviceURL: If you have a motor connected to port 1, the DeviceURL might look like this:
arduino
Copy code
vex://motor/1
Sensor DeviceURL: For a distance sensor connected to port 2, it would be:
arduino
Copy code
vex://sensor/distance/2
Controller DeviceURL: For a controller connected to port 3, the URL could be:
arduino
Copy code
vex://controller/3
Importance of DeviceURL
DeviceURL serves multiple crucial purposes:
- Communication: It enables flawless VEX Brain to linked device connection. Device URLs let developers quickly transmit commands to and gather data from particular devices.
- Organization: It helps in organizing device management within the code. Instead of dealing with arbitrary identifiers, the DeviceURL provides a clear and structured way to reference devices.
- Asynchronous Programming: Working with Node.js’s asynchronous character, DeviceURLs allow non-blocking interactions with different devices. For real-time robotics applications, where several operations might operate concurrently, this is absolutely crucial.
Setting Up Node.js for VEX Robotics
To work with DeviceURL in Node.js, you must first set up your development environment. Here’s a step-by-step guide:
1. Install Node.js
If you haven’t already, download and install Node.js from the official website. Ensure that you have the latest stable version.
2. Set Up Your Project
Create a new directory for your VEX Robotics project:
bash
Copy code
mkdir vex-robotics
cd vex-robotics
npm init -y
This commands a fresh Node.js project and generates a package.json file.
3. Install VEX Libraries
To interact with the VEX Brain, you’ll need to install the necessary libraries. For VEX, this is often done through the VEX Robotics API for Node.js.
bash
Copy code
npm install vex-robotics
4. Connect to the VEX Brain
Before using DeviceURL, establish a connection to the VEX Brain. Here’s an example code snippet to get you started:
javascript
Copy code
const Vex = require(‘vex-robotics’);
const vex = new Vex();
vex.connect(‘192.168.0.1’); // Replace with your VEX Brain’s IP address
Using DeviceURL in Node.js
Once you have the setup ready and the connection established, you can begin to use DeviceURL to interact with the devices connected to your VEX Brain.
1. Accessing Motors
To control a motor using its DeviceURL, you can write functions that leverage the DeviceURL format.
Example: Controlling a Motor
javascript
Copy code
const motorURL = ‘vex://motor/1’; // Motor connected to port 1
async function setMotorSpeed(speed) {
try {
await vex.setMotorSpeed(motorURL, speed);
console.log(`Motor speed set to ${speed}`);
} catch (error) {
console.error(‘Error setting motor speed:’, error);
}
}
// Set motor speed to 100
setMotorSpeed(100);
2. Reading Sensor Values
You can also read values from sensors connected to the VEX Brain. Using the DeviceURL for a distance sensor, you can implement a function to get the distance measurement.
Example: Reading a Distance Sensor
javascript
Copy code
const distanceSensorURL = ‘vex://sensor/distance/2’; // Distance sensor connected to port 2
async function getDistance() {
try {
const distance = await vex.readSensorValue(distanceSensorURL);
console.log(`Distance: ${distance} cm`);
} catch (error) {
console.error(‘Error reading distance:’, error);
}
}
// Get the distance measurement
getDistance();
3. Responding to Controller Input
In robotics, responding to user inputs from a controller is crucial. Using the DeviceURL for a controller, you can monitor button presses or joystick movements.
Example: Handling Controller Input
javascript
Copy code
const controllerURL = ‘vex://controller/3’; // Controller connected to port 3
async function checkController() {
try {
const buttons = await vex.getControllerButtons(controllerURL);
if (buttons[‘A’]) {
console.log(‘Button A is pressed’);
await setMotorSpeed(100); // Set motor to full speed
} else {
await setMotorSpeed(0); // Stop the motor
}
} catch (error) {
console.error(‘Error checking controller input:’, error);
}
}
// Continuously check the controller input
setInterval(checkController, 100); // Check every 100ms
Best Practices for Using DeviceURL
When programming with DeviceURL in Node.js for VEX Robotics, consider the following best practices:
1. Error Handling
Always implement error handling when interacting with devices. This ensures that your robot can gracefully recover from any unexpected situations.
2. Optimize Performance
Minimizing the VEX Brain’s requests can help you to maintain efficient codes. If at all possible, think about employing event-driven programming rather than continuously asking for sensor values.
3. Document Your Code
DeviceURL’s structured approach makes sure you record in your code the reason behind every URL and the related device. This will help you or anybody else later to grasp your project.
4. Test Regularly
During development, regularly test your code on the actual hardware. This will help identify any issues early on and ensure your robot operates as intended.
5. Use Comments for Clarity
Use comments generously to explain complex logic or critical sections of your code, especially where DeviceURLs are being utilized. This will aid in maintaining the codebase over time.
Conclusion
Anyone hoping to program the VEX Brain with Node.js must first understand and use DeviceURL. Using DeviceURL’s disciplined approach helps developers effectively handle communications with several devices, hence improving the performance of their robotic systems. DeviceURL offers a simple and efficient means of interacting with the VEX Robotics environment whether your needs are motor control, sensor value reading, or controller user input response.
Keep in mind the best practices described in this article as you start your path with VEX Robotics and Node.js. Good coding; perhaps, your robots will accomplish amazing tasks!
FAQs
- What is DeviceURL in VEX Robotics? DeviceURL is a unique identifier used to reference specific devices connected to the VEX Brain, allowing developers to interact with them programmatically.
- How do I connect to the VEX Brain using Node.js? You can connect to the VEX Brain using the VEX Robotics API for Node.js by specifying the IP address of the brain in your code.
- Can I control multiple motors using DeviceURL? Yes, each motor can be controlled individually using its unique DeviceURL, allowing for precise control in your robotic applications.
- How do I handle errors when using DeviceURL? Implement error handling using try-catch blocks in your asynchronous functions to gracefully manage any errors that arise during device interactions.
- Is it necessary to document DeviceURLs in my code? Yes, documenting DeviceURLs and their associated devices will help make your code more understandable and maintainable for yourself and others in the future.